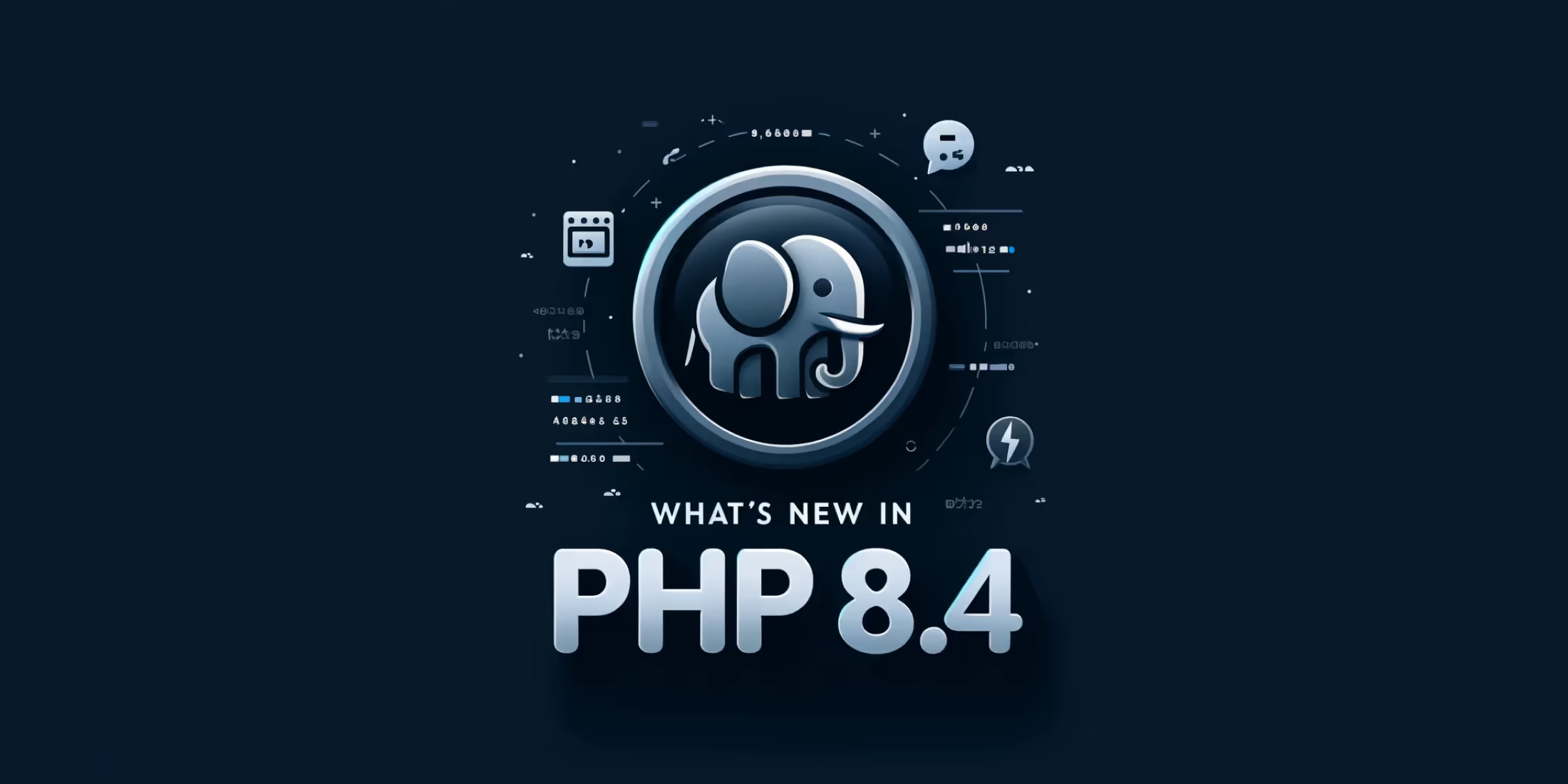
"PHP 8.4 Released with New Array Find Functions, Property Hooks, Simplified Class Instantiation, and More"
# New Array Find Functions in PHP 8.4
PHP 8.4 introduces new array find functions, including: - `array_find()` - `array_find_key()` - `array_any()` - `array_all()`
PHP Property Hooks
Property hooks in PHP are inspired by languages like Kotlin, C#, and Swift. The syntax includes two variants, resembling short and multi-line closures.
class User implements Named
{
private bool $isModified = false;
public function __construct(
private string $first,
private string $last
) {}
public string $fullName {
// Override the "read" action with arbitrary logic.
get => $this->first . " " . $this->last;
// Override the "write" action with arbitrary logic.
set {
[$this->first, $this->last] = explode(' ', $value, 2);
$this->isModified = true;
}
}
}
Property hooks will help remove boilerplate of property getters and setters, allowing a property to define access and updates using hooks.
New MyClass()->method()
Syntax Without Parentheses
Previously, when using member access during instantiation, it was required to wrap the new MyClass()
call in parentheses, or a parse error would occur. The proposed new syntax allows you to access constants, properties, and methods without the need for extra parentheses:
// Wrapping parentheses are required to access class members
$request = (new Request())->withMethod('GET')->withUri('/hello-world');
// PHP Parse error (<= PHP 8.3): syntax error, unexpected token "->"
This update addresses a minor issue, making class member access simpler by eliminating the need for surrounding parentheses or a static constructor method. The syntax change also brings PHP closer in line with other C-based languages like Java, C#, and TypeScript, which don't require parentheses around member access.
Create a DateTime from a Unix Timestamp
In PHP 8.4, creating a DateTime from a Unix timestamp becomes more convenient with the new createFromTimestamp()
method. This method supports both standard Unix timestamps and timestamps with microseconds.
$dt = DateTimeImmutable::createFromTimestamp(1718337072);
$dt->format('Y-m-d'); // 2024-06-14
$dt = DateTimeImmutable::createFromTimestamp(1718337072.432);
$dt->format('Y-m-d h:i:s.u'); // 2024-06-14 03:51:12.432000
In earlier versions of PHP, there are a few ways to create a DateTime instance from a timestamp, such as the createFromFormat()
method:
$dt = DateTimeImmutable::createFromFormat('U', (string) 1718337072);
// DateTimeImmutable @1718337072 {#7948
// date: 2024-06-14 03:51:12.0 +00:00,
// }
$dt = DateTimeImmutable::createFromFormat('U.u', (string) 1718337072.432);
// DateTimeImmutable @1718337072 {#7950
// date: 2024-06-14 03:51:12.432 +00:00,
// }
New mb_
Functions in PHP 8.4
PHP 8.4 introduces multi-byte string support for common functions like trim
, ltrim
, rtrim
, ucfirst
, and lcfirst
with the new mb_
variants:
mb_trim()
mb_ltrim()
mb_rtrim()
mb_ucfirst()
mb_lcfirst()
Asymmetric Property Visibility
Starting in PHP 8.4, properties may also have their visibility set asymmetrically with a different scope for reading and writing
class Book
{
public function __construct(
public private(set) string $title,
public protected(set) string $author,
protected private(set) int $pubYear,
) {}
}
class SpecialBook extends Book
{
public function update(string $author, int $year): void
{
$this->author = $author; // OK
$this->pubYear = $year; // Fatal Error
}
}
$b = new Book('How to PHP', 'Peter H. Peterson', 2024);
echo $b->title; // How to PHP
echo $b->author; // Peter H. Peterson
echo $b->pubYear; // Fatal Error